API Testing with Node.js and Gitlab
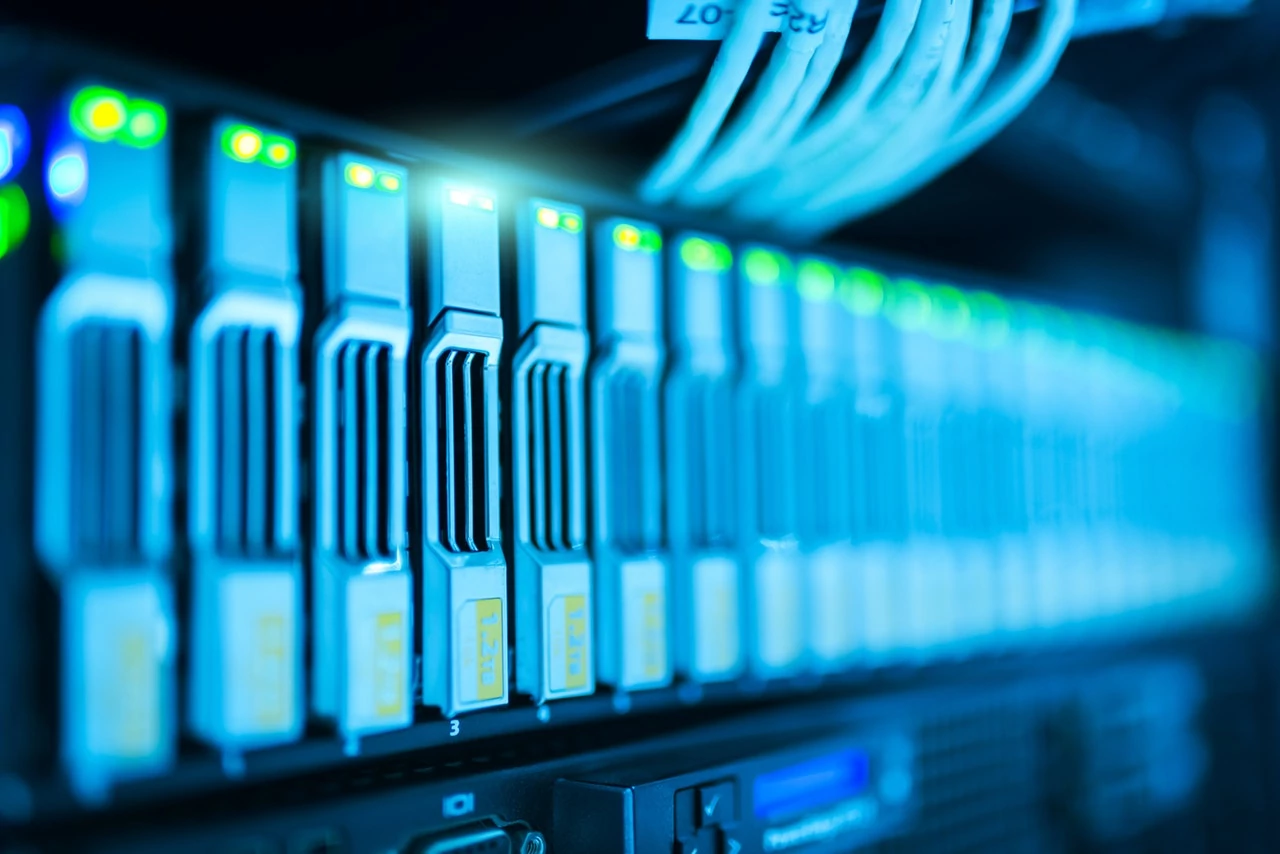
TL;DR - clone this
Having automated tests is a good thing, but having automated tests that run as part of your build process is even better. The following few paragraphs are going to illustrate how to get started down that path.
First, a few essentials and a little about the tools that I’m using here. There are quite a few continuous integration tools out there, but for this example we are going with Gitlab. There are a lot of advantages to using Gitlab, a few big ones being that it can be a source control repository, a container registry, and a build tool all in one. The fact that they have a great free tier doesn’t hurt either. Next, for writing the tests, I’m going with Node.js. It’s fast to develop in, popular as a language, and just so happens to be the language that I’ve been using the most lately.
The Test
First we need an API to test against. For that, there is an awesome free API out there for testing, JSONPlaceholder. Shout out to the dev or devs behind this, it is a great free tool for testing/ learning etc.
Now that we have an API to test, we can write some tests. For this example I’m keeping it simple, no need to go crazy here as the end goal is to illustrate this as a system, not show how to write tests. With Node.js, there are a few different HTTP request libraries out there, for this example I’m using Supertest. It’s pretty straightforward and their docs are solid. For assertions, I’m using Mochajs.
npm install supertest
npm install mocha
So two calls, a few asserts, and done in twenty-four lines of code.
const request = require('supertest')('https://jsonplaceholder.typicode.com');
const assert = require('assert');
describe('GET /todos/1', () => {
it('should work for /todos', async () => {
const response = await request
.get('/todos/1')
.expect(200);
assert.equal(response.body.userId, '1');
assert.equal(response.body.id, '1');
assert.equal(response.body.completed, false);
});
});
describe('GET /users', () => {
it('should work for /users', async () => {
const response = await request
.get('/users')
.expect(200);
assert.equal(response.body[0].name, 'Leanne Graham');
assert.equal(response.body[0].username, 'Bret');
assert.equal(response.body[0].website, 'hildegard.org');
});
});
To run the tests, you can run this command from the root of the project directory.
./node_modules/.bin/mocha —reporter spec
Or, even better, add it as a script in the package.json.
”scripts”: {
“test”: “./node_modules/.bin/mocha —reporter spec”
}
Now it’s just a:
npm run test
Pipeline
Now for the fun part, let’s make this run in Gitlab. Pipelines are setup/ managed by a .gitlab-ci.yml file. For this example we only really need to define one stage. The file should look something like this:
stages:
- testOne
testOne:
stage: testOne
image: node:latest
script:
- npm install
- npm run test
Now if you commit this to your own repo in Gitlab, you should be able to navigate to CI/CD/Pipelines and see your stage running.
Gitlab has some excellent documentation on this here, and I highly recommend checking those out. There is a lot there, but it’s a great resource.
Ok, so at this point we have Node.js running a few API tests from a Gitlab pipeline. This is pretty cool, but really it’s just scratching the surface of what you can do with Gitlab and automation. If you’ve cloned the repo for this example, you are going to notice a few extras in there, specifically the dockerfile and some additional lines in the .gitlab-ci.yml. Just ignore those for now, I’ll be discussing those in a future post.